Programming Languages: is a formalized and structured means of communicating instructions to a computer. It serves as an intermediary between human programmers and the computer’s hardware, allowing developers to write, understand, and manage code to perform various tasks and solve problems. Programming languages are designed with a specific syntax and semantics, enabling programmers to express algorithms and logic in a way that can be executed by a computer.
Types of Programming Languages
Programming languages can be categorized into several types or paradigms based on their features, design philosophy, and the way they approach problem-solving.
Here are some of the main types of programming languages:
- Imperative Languages:
– Procedural Languages: These languages focus on procedures or functions and the sequence of statements to perform tasks. Examples include C, Pascal, and Fortran.
– Object-Oriented Languages: Object-oriented languages like Java, C++, and Python use objects to encapsulate data and behavior.
– Scripting Languages: Scripting languages like JavaScript, Python, and Ruby are often used for automation and web development. - Functional Languages:
– Pure Functional Languages: These languages, such as Haskell and Lisp, emphasize immutability, first-class functions, and mathematical approaches to problem-solving.
– Multi-Paradigm Languages: Some languages, like Python and JavaScript, support both imperative and functional programming. - Declarative Languages:
– SQL (Structured Query Language): SQL is used for database querying and manipulation. It focuses on what data to retrieve rather than how to retrieve it.
– HTML/CSS: While not traditional programming languages, HTML and CSS are declarative languages for structuring and styling web content. - Scripting Languages:
– Shell Scripting Languages: Shell scripts (e.g., Bash and PowerShell) are used for automating system-level tasks and interacting with the operating system.
– Web Scripting Languages: Languages like PHP and Perl are used for server-side scripting in web development. - Markup Languages:
– HTML (Hypertext Markup Language): HTML is used for structuring content on the web.
– XML (Extensible Markup Language): XML is used for defining document structures and data interchange. - Domain-Specific Languages (DSLs):
– Embedded DSLs: DSLs integrated into a host language for specific tasks, such as regular expressions in Python or SQL in Java.
– Standalone DSLs: Independent languages designed for specific domains, like VHDL for hardware description or SQL for database queries. - Concurrency and Parallel Languages:
– Languages with Concurrency Support: Go and Erlang are designed for building concurrent and distributed systems.
– Parallel Processing Languages: Languages like CUDA and OpenMP are used for parallel computing. - Logic Programming Languages:
– Prolog: Prolog is a logic programming language used for symbolic reasoning and rule-based problem-solving. - Assembly Languages:
– Low-Level Assembly Languages: These languages are specific to a particular computer architecture and are used for system programming and hardware interaction. - Array Languages:
– MATLAB: MATLAB is used for numerical and matrix operations in scientific and engineering applications.
– NumPy (for Python): NumPy provides similar functionality for numerical computing in Python. - Natural Language Programming Languages:
– These languages aim to enable programming through natural language or human-like instructions, although they are still experimental and not widely used. - Visual Programming Languages:
– These languages use graphical elements and drag-and-drop interfaces for programming. Examples include Scratch and Blockly. - Compiled vs. Interpreted Languages:
– Programming languages can also be categorized based on whether they are compiled (e.g., C++) or interpreted (e.g., Python). Compiled languages are translated into machine code before execution, while interpreted languages are executed line by line by an interpreter. - Static vs. Dynamic Typing:
– Languages can be classified based on their typing systems. Static typing (e.g., C, Java) requires variable types to be declared at compile-time, while dynamic typing (e.g., Python, JavaScript) allows types to be determined at runtime. - High-Level vs. Low-Level Languages:
– High-level languages (e.g., Python, Ruby) provide abstractions that simplify programming, while low-level languages (e.g., Assembly, C) offer more direct control over hardware but require more complex code. - Cross-Platform Languages:
– Some languages, like Java, Kotlin, and JavaScript, are designed to run on multiple platforms with minimal modifications. - Esoteric Languages:
– These are languages designed for fun or experimental purposes and often have unusual syntax and features. Examples include Brainfuck and LOLCODE.
The choice of programming language depends on the specific requirements of a project, the domain of application, performance needs, and the developer’s familiarity with the language. Many modern languages are also multi-paradigm, incorporating features from multiple types to provide flexibility and versatility.
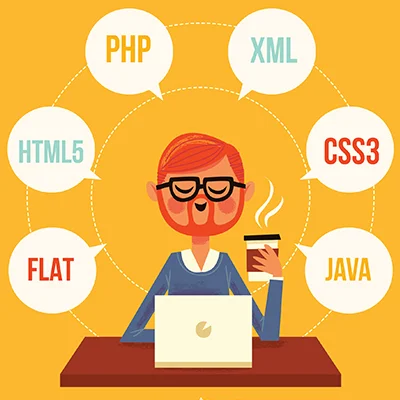
List of Programming Languages
There are numerous programming languages, each designed for specific purposes and with unique features.
Here’s a list of some well-known programming languages
- Python: Known for its simplicity and readability, Python is widely used in web development, data analysis, machine learning, and more.
- JavaScript: A versatile language used for web development, allowing for interactive web pages and server-side scripting (Node.js).
- Java: A popular language for building cross-platform applications, including web applications, Android apps, and enterprise software.
- C++: An extension of C, C++ is used for system programming, game development, and high-performance applications.
- C : A fundamental language used for system programming, embedded systems, and low-level operations.
- C# : Developed by Microsoft, C# is primarily used for Windows application development and game development with Unity.
- Ruby: Known for its elegant syntax, Ruby is used in web development (Ruby on Rails) and scripting.
- Swift: Developed by Apple, Swift is used for iOS, macOS, watchOS, and tvOS app development.
- Kotlin: Another language for Android app development, Kotlin is interoperable with Java and offers concise syntax.
- Go (Golang): Created by Google, Go is known for its speed and efficiency, making it suitable for web servers and system tools.
- Rust: Known for its memory safety features, Rust is used for system-level programming and applications where performance and security are critical.
- PHP: A server-side scripting language used for web development and building dynamic web applications.
- SQL: Not a general-purpose programming language but used for database management and querying.
- TypeScript: A statically typed superset of JavaScript, TypeScript adds type checking and other features to JavaScript.
- Perl: Known for its text processing capabilities, Perl is used for system administration and web development.
- Haskell: A functional programming language known for its strong type system and mathematical foundations.
- Scala: Combining object-oriented and functional programming, Scala is used for web development and data analysis.
- R : Specifically designed for statistical computing and data analysis, R is widely used in data science.
- Lua: Known for its embeddability, Lua is used in game development (e.g., in the popular game engine, Unity) and scripting.
- Dart: Developed by Google, Dart is used for web and mobile app development, particularly for the Flutter framework.
- Groovy: An object-oriented scripting language that runs on the Java Virtual Machine (JVM) and is often used for automation and testing.
- Elixir: A functional, concurrent language designed for building scalable and fault-tolerant applications, especially in the realm of web development with the Phoenix framework.
- Clojure: A Lisp dialect that runs on the Java Virtual Machine (JVM) and is used for functional programming and data processing.
- Ada: A language designed for safety-critical and real-time systems, commonly used in aerospace and defense industries.
- COBOL: Initially designed for business data processing, COBOL is still in use for legacy systems in the finance and government sectors.
- Fortran: Designed for scientific and engineering computations, Fortran is known for its numerical and mathematical capabilities.
- PL/SQL: Oracle’s procedural extension to SQL, used for database development and management.
- Erlang: Developed for building fault-tolerant, distributed systems, Erlang is used in telecommunications and messaging systems.
- Julia: Designed for high-performance numerical and scientific computing, Julia is known for its speed and ease of use.
- Scratch: A visual programming language designed for teaching programming concepts to beginners, often used in educational settings.
This list is by no means exhaustive, and the popularity of programming languages may change over time. New languages may have emerged since my last update, and the usage of existing languages may have evolved.
Features of Programming Languages
Programming languages have various features and characteristics that make them suitable for different tasks and application domains.
Here are some of the key features of programming languages:
- Syntax: The syntax of a programming language defines its rules for writing code, including keywords, punctuation, and structure. Clear and consistent syntax contributes to code readability and maintainability.
- Data Types: Programming languages offer various data types, such as integers, floats, strings, and booleans, to represent different kinds of data. Some languages support dynamic typing, while others use static typing.
- Variables: Variables allow programmers to store and manipulate data in memory. Languages have rules for declaring, initializing, and using variables.
- Operators: Programming languages provide operators for performing operations on data, including arithmetic, comparison, logical, and bitwise operations.
- Control Structures: Control structures like loops (for, while, do-while) and conditional statements (if, else, switch) determine the flow of program execution.
- Functions/Methods: Functions or methods allow developers to group code into reusable blocks and pass data in and out. Functions can be built-in or user-defined.
- Object-Oriented Features: Object-oriented languages support concepts like classes, objects, inheritance, and polymorphism for organizing code into reusable and maintainable units.
- Error Handling: Programming languages provide mechanisms for handling errors and exceptions, allowing programs to gracefully recover from unexpected situations.
- Standard Libraries: Languages often come with standard libraries that offer pre-built functions and modules for common tasks, such as file I/O, network communication, and data manipulation.
- Concurrency and Parallelism: Some languages provide built-in support for managing concurrent or parallel execution of code, allowing efficient use of multi-core processors.
- Memory Management: Memory management can be automatic (e.g., garbage collection in Java) or manual (e.g., memory allocation and deallocation in C/C++).
- I/O Operations: Languages offer mechanisms for input and output operations, enabling programs to interact with files, databases, and external devices.
- Modularity: Modularity features, such as namespaces and modules, allow developers to organize code into separate, reusable units for easier maintenance and collaboration.
- Portability: The ability to run code on different platforms and architectures is crucial. Some languages are cross-platform, while others are platform-specific.
- Extensibility: Some languages support adding third-party libraries and extensions to enhance functionality and meet specific requirements.
- Interoperability: The ability to interface with code written in other languages, often through APIs or foreign function interfaces (FFIs), is important for integrating different technologies.
- Community and Ecosystem: The size and activity of a language’s community and its ecosystem of libraries, frameworks, and tools can significantly impact development productivity.
- Performance: Languages vary in terms of performance, depending on factors like compiled vs. interpreted, low-level vs. high-level, and optimization capabilities.
- Security Features: Security-oriented languages and practices help developers write secure code, preventing vulnerabilities and attacks.
- Documentation and IDE Support: Availability of documentation, integrated development environments (IDEs), and code editors can improve the development experience.
- Testing and Debugging: Languages may offer tools and features for testing code, debugging, and profiling to identify and fix issues efficiently.
- Community and Ecosystem: The size and activity of a language’s community and its ecosystem of libraries, frameworks, and tools can significantly impact development productivity.
Each programming language has its strengths and weaknesses, and the choice of language depends on the specific requirements of a project, the target platform, and the developer’s familiarity and preferences.
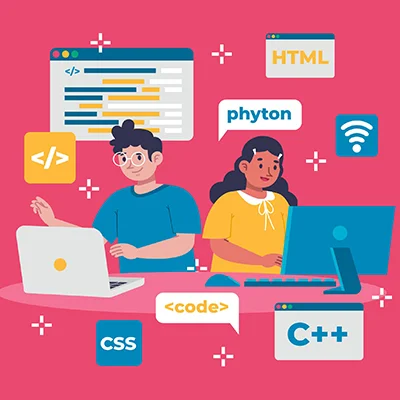
How Does it Work
Programming languages work as an intermediary between human programmers and computer hardware, enabling humans to communicate instructions to computers in a way that the machines can understand and execute.
Here’s a simplified overview of how programming languages work:
- Writing Code: Programmers write code using a text editor or integrated development environment (IDE). They use the syntax and semantics of a specific programming language to express algorithms, logic, and instructions to solve a particular problem.
- Compilation or Interpretation:
– Compiled Languages: In the case of compiled languages like C or C++, the code is saved in source code files with specific file extensions (e.g., .c or .cpp). Programmers use a compiler to translate this human-readable source code into machine code, which is a binary format that the computer’s CPU can execute directly. The resulting binary file is called an executable, which can be run independently on compatible hardware.
– Interpreted Languages: In interpreted languages like Python or JavaScript, the source code remains in human-readable form. When a program is executed, an interpreter reads and executes the code line by line, translating it into machine-level instructions on the fly. This allows for platform independence, as long as an interpreter for the language is available on the target platform. - Execution: During execution, the computer follows the instructions provided in the code. It performs calculations, reads and writes data, interacts with external devices, and performs other tasks specified in the program.
- Data Manipulation: Data is manipulated in memory using variables, data types, and operations defined in the programming language. Programmers can control the flow of execution using control structures like loops and conditional statements.
- Functions and Libraries: Programming languages often provide a way to encapsulate code into functions or methods, which can be reused throughout the program. Additionally, languages often include libraries or modules that contain pre-written code for common tasks, enabling developers to save time and effort.
- Error Handling: The language provides mechanisms for detecting and responding to errors and exceptions, allowing the program to gracefully handle unexpected situations.
- Input/Output (I/O): Programs can interact with the outside world through input (e.g., reading user input or data from files) and output (e.g., displaying information to the screen or writing data to files).
- Memory Management: Depending on the language, memory management can be handled automatically (e.g., garbage collection in languages like Java) or manually (e.g., explicit memory allocation and deallocation in C or C++).
- Concurrency and Parallelism: Some languages offer built-in support for concurrent or parallel execution, allowing programs to take advantage of multi-core processors and perform tasks concurrently.
- Portability: Programming languages differ in their degree of platform independence. Some languages are designed to run on multiple platforms without modification, while others may require specific adaptations for different operating systems or hardware architectures.
In summary, programming languages provide a structured way for humans to communicate their intent to computers. The choice of a particular language depends on factors such as the nature of the problem, performance requirements, platform compatibility, and the developer’s familiarity with the language. Once written and translated (either compiled or interpreted), the code becomes a set of instructions that the computer can execute to perform the desired tasks.
Which is most powerful programming language?
The term “most powerful” when referring to programming languages is subjective and can depend on various factors, including the context and specific use case. Different languages excel in different areas, and what may be considered powerful for one task might not be as effective for another. It’s important to choose a programming language that aligns with the requirements and goals of a particular project.
Here are a few programming languages that are often considered powerful in specific domains:
- C/C++ : C and C++ are often regarded as powerful languages because they provide low-level control over hardware and memory, making them suitable for system programming, embedded systems, and high-performance applications. They are commonly used in operating systems, game development, and real-time systems.
- Python: Python is considered powerful in the context of ease of use and versatility. It’s known for its readability, extensive standard library, and support for a wide range of domains, including web development, data analysis, machine learning, and scientific computing.
- Java: Java is considered powerful in enterprise and large-scale systems due to its strong typing, portability, and support for multi-threading. It’s widely used in server-side applications and Android app development.
- C# : C# is powerful for Windows application development, game development using Unity, and enterprise software. It offers features like strong typing, garbage collection, and support for the .NET framework.
- Rust : Rust is often seen as a powerful language for system programming and applications where both performance and safety are crucial. It provides memory safety guarantees while still allowing low-level control over resources.
- Haskell : Haskell is considered powerful in the realm of functional programming due to its advanced type system and strong support for abstract mathematical concepts. It’s used in areas like theorem proving and compiler development.
- Lisp : Lisp is known for its power in symbolic processing and artificial intelligence applications. It offers features like dynamic typing, macros, and a flexible syntax.
- Assembly Language : Assembly languages, which are specific to computer architectures, are considered powerful when it comes to the most direct control over hardware. However, they are also the most challenging to work with due to their low-level nature.
It’s crucial to note that the power of a programming language often comes with trade-offs. For example, lower-level languages like C and C++ provide greater control but may require more effort to write and maintain code, while higher-level languages like Python and Java offer productivity benefits but may not provide the same level of control over system resources. The choice of the “most powerful” language depends on the specific requirements of a project and the developer’s expertise in the language.
What is the future of programming Language ?
The future of programming languages is a dynamic and evolving landscape driven by technological advancements, changing industry needs, and developer preferences. While it’s challenging to predict the future with certainty,
Several trends and directions are likely to shape the evolution of programming languages:
- Specialized Languages: As technology continues to advance, we may see the emergence of more specialized programming languages designed for specific domains and applications. These languages will aim to optimize performance and productivity for niche use cases.
- Concurrency and Parallelism: With the increasing prevalence of multi-core processors, programming languages that provide better support for concurrent and parallel programming will gain importance. Languages like Rust and Go are already addressing this need.
- Quantum Computing Languages: As quantum computing becomes more accessible, there will be a demand for programming languages and frameworks that allow developers to harness the power of quantum computers.
- AI-Enhanced Programming: AI-driven tools and assistants will become more integrated into the programming process, helping developers with tasks like code generation, debugging, and optimization.
- Cross-Platform Development: The need for writing code that works seamlessly across different platforms (e.g., mobile, web, desktop) will continue to drive the development of languages and frameworks that simplify cross-platform development.
- Sustainability and Energy Efficiency: Energy-efficient programming and algorithms will gain importance as environmental concerns become more prominent. Languages and tools that prioritize energy efficiency will be favored.
- Low-Code and No-Code Development: The trend toward low-code and no-code development platforms will continue, making it easier for non-programmers to create applications.
- Ethical and Secure Coding: Emphasis on ethical coding practices and security will increase, leading to languages and tools that make it easier to write secure and ethical code.
- Blockchain Development: As blockchain technology evolves, specialized languages and platforms for creating smart contracts and decentralized applications (DApps) will become more relevant.
- Natural Language Programming: Advancements in natural language processing (NLP) may lead to programming languages that allow developers to interact with code using natural language commands.
- Interoperability: Languages that facilitate interoperability between different technologies and ecosystems will be in demand as integration between systems and services becomes increasingly important.
- Open Source and Community Collaboration: The open-source model and collaborative development will remain prominent, allowing for the continuous evolution of existing languages and the creation of new ones.
- Globalization: As software development becomes more global, languages and tools that support internationalization and localization will be essential.
- Education and Accessibility: Programming languages designed to simplify the learning process and make coding more accessible, especially for beginners and underrepresented groups, will continue to be developed.
- Human-Machine Collaboration: Programming languages may evolve to enable closer collaboration between humans and machines, supporting more intuitive and interactive development experiences.
It’s important to note that existing programming languages will continue to evolve and adapt to new challenges and opportunities. The choice of a programming language for a particular project will depend on the project’s requirements, the developer’s familiarity with the language, and the available tools and libraries. As the technology landscape evolves, developers should be prepared to learn and adapt to new languages and paradigms.
Cloud Computing: Definition, Types, Scope, & Benefits